Spectral Weighting Filters¶
Module name: splweighting
This module implements spectral weighting filters for the sound pressure level (SPL) in air according to [IEC-61672]. Spectral weighting is part of aucoustic measurements. It is used by sound level meters for example. The weighting functions are derived from different equal loudness contours of human hearing. The weighted levels aim to provide a better correlation to the perception of loudness.
Implemented weighting functions¶
There are three weighting functions implemented:
- A-Weighting: based on the 40-phon equal loudness contour
- B- and C-weighting: for sounds above 70 phon, (B-Weighting is not used that often)
The filter coefficient design is based on the implementation of A- and C-weighting in [R2].
The weighting functions are defined in [IEC-61672] can be described by the following equations:
The frequency responses absolute values of all implemented weighting filters can be seen in the following figure:
(Source code, png, hires.png, pdf)
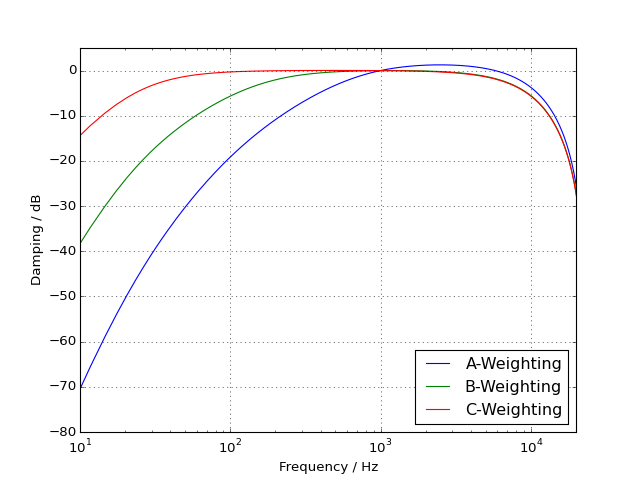
References¶
[IEC-61672] | (1, 2) Electroacoustics - Sound Level Meters (http://www.iec.ch) |
[R2] | Christophe Couvreur, MATLAB(R) implementation of weightings, http://www.mathworks.com/matlabcentral/fileexchange/69-octave, Faculte Polytechnique de Mons (Belgium) couvreur@thor.fpms.ac.be |
Functions¶
-
splweighting.
a_weighting_coeffs_design
(sample_rate)[source]¶ Returns b and a coeff of a A-weighting filter.
Parameters: sample_rate : scalar
Sample rate of the signals that well be filtered.
Returns: b, a : ndarray
Filter coefficients for a digital weighting filter.
See also
b_weighting_coeffs_design
- B-Weighting coefficients.
c_weighting_coeffs_design
- C-Weighting coefficients.
weight_signal
- Apply a weighting filter to a signal.
scipy.lfilter
- Filtering signal with b and a coefficients.
Examples
>>> b, a = a_weighting_coeff_design(sample_rate)
To Filter a signal use scipy lfilter:
>>> from scipy.signal import lfilter >>> y = lfilter(b, a, x)
-
splweighting.
b_weighting_coeffs_design
(sample_rate)[source]¶ Returns b and a coeff of a B-weighting filter.
B-Weighting is no longer described in DIN61672.
Parameters: sample_rate : scalar
Sample rate of the signals that well be filtered.
Returns: b, a : ndarray
Filter coefficients for a digital weighting filter.
See also
a_weighting_coeffs_design
- A-Weighting coefficients.
c_weighting_coeffs_design
- C-Weighting coefficients.
weight_signal
- Apply a weighting filter to a signal.
Examples
>>> b, a = b_weighting_coeff_design(sample_rate)
To Filter a signal use :function: scipy.lfilter:
>>> from scipy.signal import lfilter >>> y = lfilter(b, a, x)
-
splweighting.
c_weighting_coeffs_design
(sample_rate)[source]¶ Returns b and a coeff of a C-weighting filter.
Parameters: sample_rate : scalar
Sample rate of the signals that well be filtered.
Returns: b, a : ndarray
Filter coefficients for a digital weighting filter.
See also
a_weighting_coeffs_design
- A-Weighting coefficients.
b_weighting_coeffs_design
- B-Weighting coefficients.
weight_signal
- Apply a weighting filter to a signal.
Examples
b, a = c_weighting_coeffs_design(sample_rate)
To Filter a signal use scipy lfilter:
from scipy.signal import lfilter y = lfilter(b, a, x)
-
splweighting.
plot_weightings
()[source]¶ Plots all weighting functions defined in :module: splweighting.
-
splweighting.
weight_signal
(data, sample_rate=44100, weighting='A')[source]¶ Returns filtered signal with a weighting filter.
Parameters: data : ndarray
Input signal to be filtered.
sample_rate : int
Sample rate of the signal.
weighting : {‘A’, ‘B’, ‘C’}
Specify the weighting function by a string.
Returns: outdata : ndarray
Filtered output signal. The output will be weighted by the specified filter function.