Gammatone Filter Bank¶
Module name: gammatone
This module implements gammatone filters a filtering routine and a filterbank class.
The filterbank is based on [Hohmann2002].
(Source code, png, hires.png, pdf)
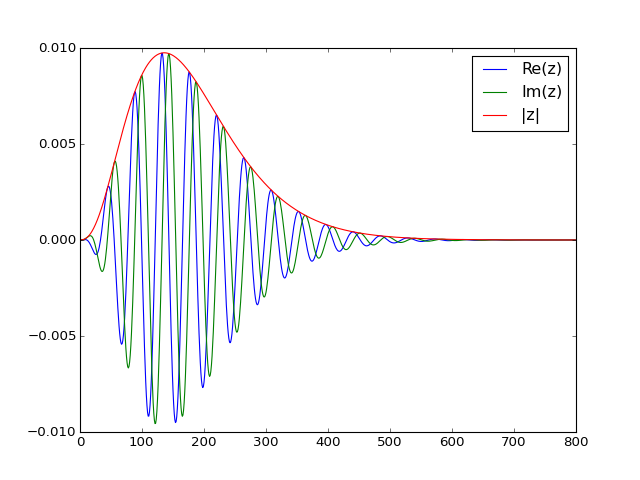
Examples¶
- TODO:
- Tests,
- nice introduction with example,
References¶
[Hohmann2002] | (1, 2, 3, 4, 5) Hohmann, V., Frequency analysis and synthesis using a Gammatone filterbank, Acta Acustica, Vol 88 (2002), 433–442 |
Functions¶
-
class
gammatone.
GammatoneFilterbank
(samplerate=44100, order=4, startband=-12, endband=12, normfreq=1000.0, density=1.0, bandwidth_factor=1.0, desired_delay_sec=0.02)[source]¶ Returns a GammatoneFilterbank instance for filtering signals.
Parameters: samplerate : scalar
Default: 44100.
order : scalar
Default: 4.
startband : scalar
Default: -12,
endband : scalar
Default: 12,
normfreq : scalar
Default: 1000.0,
density : scalar
Default: 1.0,
bandwidth_factor : scalar
Default: 1.0,
desired_delay_sec : scalar
Default: 0.02
Methods
-
analyze
(signal, states=None)[source]¶ Returns a generator yielding filtered signal bands and states.
-
estimate_max_indices_and_slopes
(delay_samples=None)[source]¶ Returns Estimate of maximum index and slopes at max. Used for estimation of phase-factors for delaying bands.
-
freqz
(nfft=4096, plotfun=None)[source]¶ Returns frequency and impulse responses. Accepts plotfun function for cusomized plotting.
-
init_delay
(desired_delay_sec)[source]¶ Initializes delay estimation and variables for delay bands to achieve a optimized impulse response.
-
init_gains
()[source]¶ Initializes gains for weighting bands leading to a equalized freqresponse when summing (synthesizing) bands.
-
-
gammatone.
design_filtbank_coeffs
(samplerate, order, centerfrequencies, bandwidths=None, bandwidth_factor=None, attenuation_half_bandwidth_db=-3)[source]¶ Designs complex coefficients for a gammatone filter bank.
Parameters: samplerate : int
order : int
centerfrequencies : arraylike
bandwidths : arraylike (optional)
bandwidth_factor : scalar (1.0 is auditory erb)
attenuation_half_bandwidth_db : scalar
Returns: generator of b, a coefficients
-
gammatone.
design_filter
(sample_rate=44100, order=4, centerfrequency=1000.0, band_width=None, band_width_factor=1.0, attenuation_half_bandwidth_db=-3)[source]¶ Returns filter coefficient of a gammatone filter [Hohmann2002].
Parameters: sample_rate : int/scalar
order : int
centerfrequency : scalar
band_width : scalar
band_width_factor : scalar
attenuation_half_bandwidth_db : scalar
Returns: b, a : ndarray, ndarray
-
gammatone.
erb_aud
(centerfrequency)[source]¶ Retrurns equivalent rectangular band width of an auditory filter. Implements Equation 13 in [Hohmann2002].
Parameters: centerfrequency : scalar /Hz
The center frequency in Hertz of the desired auditory filter.
Returns: erb : scalar
Equivalent rectangular bandwidth of an auditory filter at centerfrequency.
-
gammatone.
erb_count
(centerfrequency)[source]¶ Returns the equivalent rectangular band count up to centerfrequency.
Parameters: centerfrequency : scalar /Hz
The center frequency in Hertz of the desired auditory filter.
Returns: count : scalar
Number of equivalent bandwidths below centerfrequency.
-
gammatone.
erbscale_to_hertz
(erb)[source]¶ Returns frequency in Hertz from ERB value. Implements Equation 17 in [Hohmann2002].
Parameters: erb : scalar
The corresponding value on the ERB-Scale.
Returns: frequency : scalar
The Frequency in Hertz.
-
gammatone.
fosfilter
(b, a, order, signal, states=None)[source]¶ Return signal filtered with b and a (first order section) by filtering the signal order times.
This Function was created for filtering signals by first order section cascaded complex gammatone filters.
Parameters: b, a : ndarray, ndarray
Filter coefficients of a first order section filter. Can be complex valued.
order : int
Order of the filter to be applied. This will be the count of refiltering the signal order times with the given coefficients.
signal : ndarray
Input signal to be filtered.
states : ndarray, default None
Array with the filter states of length order. Initial you can set it to None.
Returns: signal : ndarray
Output signal, that is filtered and complex valued (analytical signal).
states : ndarray
Array with the filter states of length order. You need to loop it back into this function when block processing.
-
gammatone.
frequencies_gammatone_bank
(start_band, end_band, norm_freq, density)[source]¶ Returns centerfrequencies and auditory Bandwidths for a range of gamatone filters.
Parameters: start_band : int
Erb counts below norm_freq.
end_band : int
Erb counts over norm_freq.
norm_freq : scalar
The reference frequency where all filters are around
density : scalar
ERB density 1would be erb_aud.
Returns: centerfrequency_array : ndarray
-
gammatone.
freqz_fos
(b, a, order, nfft, plotfun=None)[source]¶ Returns Frequency Response and impulse response of first order section coeffs.
Parameters: b : arraylike
a : arraylike
order : int
nfft : int
plotfun : function(frequencies, response)
Returns: freqresponse : arraylike
frequencies : arraylike
response : arraylike
-
gammatone.
hertz_to_erbscale
(frequency)[source]¶ Returns ERB-frequency from frequency in Hz. Implements Equation 16 in [Hohmann2002].
Parameters: frequency : scalar
The Frequency in Hertz.
Returns: erb : scalar
The corresponding value on the ERB-Scale.